Let's be honest, performing per-environment builds just to get your .env configuration into the package is painful. Not to mention, it slows down your build and release pipelines considerably. It is a "popular" antipattern seen in React builds.
Let's talk about a simple strategy & hook that you can use to simplify your configuration and allow you to have a single build for Dev/QA/UAT/Prod deployments.
Dynamic Configuration: Setting Things Up
The strategy we'll be using is to use the hostnames of your destination infrastructure to select which configuration is applicable.
For example, you might have the following hostnames in your application:
With this information, our app can cleanly detect which environment it's in and select the configuration accordingly. Now, your environment hostnames (URLs) are going to differ, so your method of selection will vary, but the concepts remain the same:
Select your config based on the environment.
The Hook: Accessing Your Configuration
Let's get down to the code itself. It should only take a few minutes to set up properly.
1. Add a directory structure that matches the screenshot below:
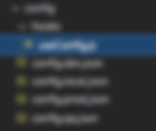
2. Add your configuration for each of your environments in the config.*.json files.
3. Open useConfig.js and add the following code:
import localConfig from '../config.local.json';
import devConfig from '../config.dev.json';
import qaConfig from '../config.qa.json';
import prodConfig from '../config.prod.json';
const isEnv = prefix => {
const hostname = window.location.hostname;
return hostname.indexOf(prefix) > -1;
};
const useConfig = () => {
// TODO: Update these to match your hostnames
const isLocalhost = isEnv('localhost');
const isDev = isEnv('dev');
const isQa = isEnv('qa');
const isProd = isEnv('contoso.com');
if (isLocalhost) {
return localConfig;
} else if (isDev) {
return devConfig;
} else if (isQa) {
return qaConfig;
} else if (isProd) {
return prodConfig;
}
};
export default useConfig;
4. You're done! with your app dynamically selecting which config to use at runtime, you
no longer need to run an npm or yarn build per environment.
Summary
All in all, this is a simple solution that will get you to a place where it's easy to draft environment configuration without having to worry about multiple builds in react. This same concept applies to Angular, Vue.js, etc.
I should note that the code above is not production-ready. Also, if you have concerns regarding bundling all of your configuration files in the deployment package, set up your deployment pipelines to delete the irrelevant config prior to deploying your production package.